https://docs.aws.amazon.com/ko_kr/rekognition/latest/dg/labels-detect-labels-image.html
ìŽë¯žì§ìì ë ìŽëž ê°ì§ - Amazon Rekognition
êž°ê³ ë²ììŒë¡ ì ê³µëë ë²ìì ëë€. ì ê³µë ë²ì곌 ì볞 ììŽì ëŽì©ìŽ ìì¶©íë 겜ì°ìë ììŽ ë²ì ìŽ ì°ì í©ëë€. ìŽë¯žì§ìì ë ìŽëž ê°ì§ ìŽ DetectLabelsìì ì ì¬ì©íì¬ ìŽë¯žì§ìì ë ìŽëž (
docs.aws.amazon.com
- ë©ëŽìŒì ì°žê³ íë€.
ìë륌 ì°žê³ íì¬ ë²í·ì ë§ë ë€.
https://msdev-st.tistory.com/155
[AWS] S3 ë²í· ë§ë€êž° _ ì€í ëŠ¬ì§ ë§ë€ìŽì ì¬ì©íêž°
S3 ë²í· ë§ë€êž° _ ì€í ëŠ¬ì§ ë§ë€ìŽì ì¬ì©íêž°AWSì S3ë? Simple Storage Serviceì ìœìë¡ íìŒ ìë²ì ìí ì íë ìë¹ì€ë€. ìŒë°ì ìž íìŒìë²ë ížëíœìŽ ìŠê°íšì ë°ëŒì ì¥ë¹ë¥Œ ìŠì€íë ì
msdev-st.tistory.com
awsì IAMìì, AmazonRekognitionFullAcess ê¶íì¶ê°íêž°
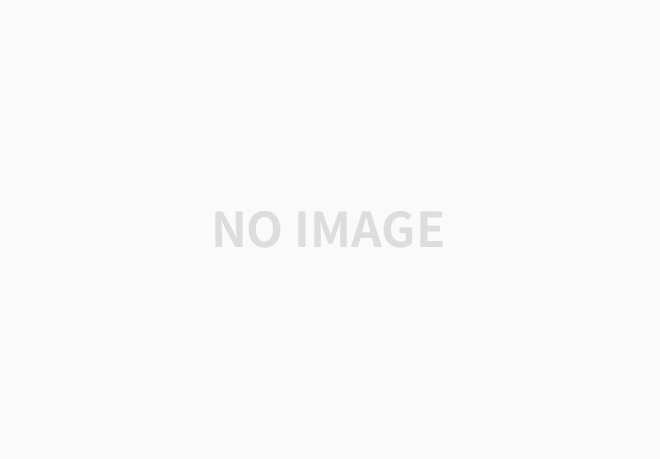
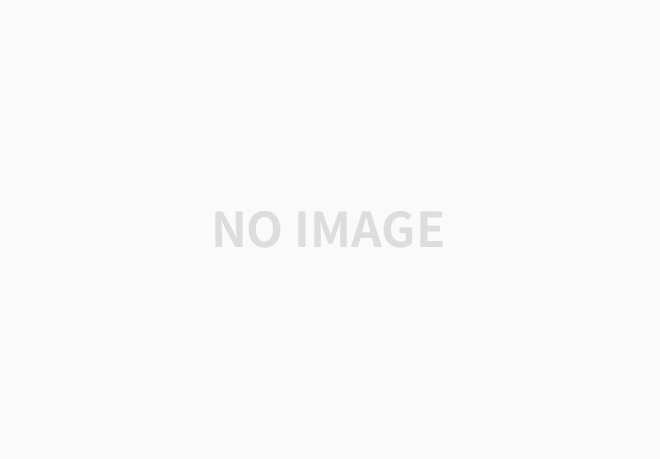
serverlessë¡ ë§ë íŽë륌 vscodeë¡ ì°ë€.
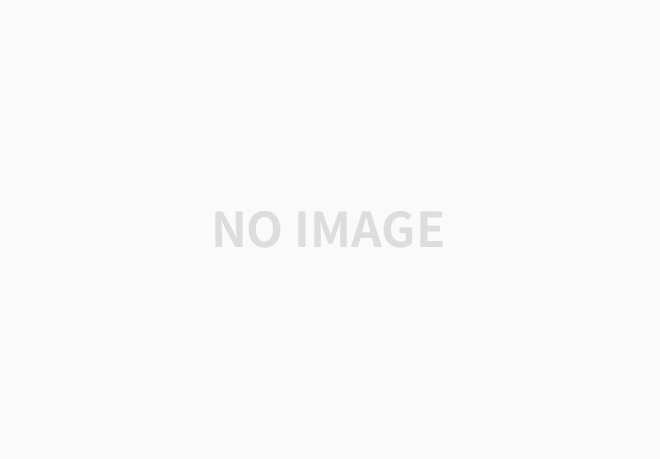
ë²í· ë§ë€ ë ì¬ì©í ìŽëŠì ë£ìŒë©Ž ëë€.
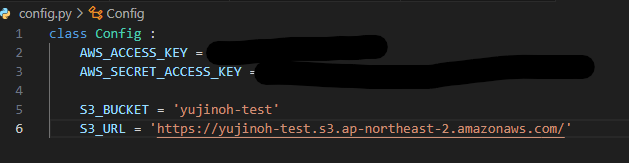
app.py ìì±
from flask import Flask
from flask_restful import Api
from resources.image import FileUploadResource
app = Flask(__name__)
api = Api(app)
# 겜ë¡ì 늬ìì€ë¥Œ ì°ê²°íë€.
api.add_resource( FileUploadResource , '/upload' )
if __name__ == '__main__' :
ââââapp.run()
resources íŽë ìì image.py ìì±
from flask_restful import Resource
from flask import request
from datetime import datetime
import boto3
from config import Config
class FileUploadResource(Resource) :
ââââdef post(self):
ââââââââ# 1. íŽëŒìŽìžížë¡ë¶í° ë°ìŽí°ë¥Œ ë°ììšë€.
ââââââââ# íìŒì request.files ìì ìê³ ,
ââââââââ# í
ì€ížë request.form ìì ìë€.
ââââââââif 'photo' not in request.files :
ââââââââââââreturn {"result":"fail", "error":"íìŒì ì
ë¡ë íìžì."}, 400
ââââââââ
ââââââââif 'content' not in request.form :
ââââââââââââreturn {"result":"fail", "error":"ëŽì©ì ìì± íìžì."}, 400
ââââââââ
ââââââââfile = request.files.get('photo')
ââââââââ
ââââââââif 'image' not in file.content_type :
ââââââââââââreturn {'result':'fail',
ââââââââââââââââââââ'error':'ìŽë¯žì§ íìŒë§ ì
ë¡ë ê°ë¥í©ëë€'}, 400
ââââââââ
ââââââââprint(file)
ââââââââcontent = request.form['content']
ââââââââprint(content)
ââââââââ# íìŒì s3ì ì
ë¡ë íŽìŒ íëë°,
ââââââââ# 뚌ì , íìŒëª
ì ì ëí¬ íŽìŒ íë€.
ââââââââ# ë°ëŒì, ì ëí¬í íìŒëª
ìŒë¡ ë°ê¿ì ì
ë¡ë íë€.
ââââââââ# íì¬ìê°ê³Œ ì ì ììŽë ë±ì ì¡°í©íŽì ë§ë ë€.
ââââââââcurrent_time = datetime.now()
ââââââââfile_name = current_time.isoformat().replace(':','_') + '.' + file.content_type.split('/')[-1]
ââââââââprint(file_name)
ââââââââ# ì ì ê° ì
ë¡ëí íìŒëª
ì, ëŽê° ë§ë íìŒëª
ìŒë¡ ë°êŸŒë€.
ââââââââfile.filename = file_name
ââââââââ# s3ì íìŒì ì
ë¡ëíë€.
ââââââââ# awsì ìë¹ì€ë€ì íìŽì¬ìœëë¡ ìì± í ì ìë
ââââââââ# boto3 ëŒìŽëžë¬ëŠ¬ë¥Œ ìŽì©íŽì ìœë륌 ìì±íë€.
ââââââââ# ( ì€ì¹ë, $ pip install boto3 )
ââââââââclient = boto3.client('s3' ,
âââââââââââââââââââââââââââââââaws_access_key_id = Config.AWS_ACCESS_KEY,
âââââââââââââââââââââââââââââââaws_secret_access_key = Config.AWS_SECRET_ACCESS_KEY)
ââââââââ# ExtraArgs = {'ACL':'public-read','ContentType':'image/jpg'} ì€ìë¶ë¶
ââââââââtry :
ââââââââââââclient.upload_fileobj(file,
ââââââââââââââââââââââââââââââââââConfig.S3_BUCKET,
ââââââââââââââââââââââââââââââââââfile_name,
ââââââââââââââââââââââââââââââââââExtraArgs = {'ACL':'public-read',
âââââââââââââââââââââââââââââââââââââââââââââââ'ContentType':file.content_type})
ââââââââexcept Exception as e:
ââââââââââââreturn {"result":"fail", "error":str(e)}, 500
ââââââââreturn {"result":"success", "url":Config.S3_URL + file_name}
vscode cmdìì ì€ì¹íë€.
pip install boto3
rekognition.py ìì±
from flask import request
from flask_restful import Resource
from datetime import datetime
import boto3
from config import Config
class ObjectDetectionResource(Resource):
ââââdef post(self):
ââââââââif 'photo' not in request.files :
ââââââââââââreturn {"result":"fail", "error":"ì¬ì§ì íìì
ëë€."}, 400
ââââââââ
ââââââââfile = request.files['photo']
ââââââââif 'image' not in file.content_type :
ââââââââââââreturn {"result":"fail", "error":"ìŽë¯žì§íìŒì ì
ë¡ë íìžì."}, 400
ââââââââ
ââââââââcurrent_time = datetime.now()
ââââââââfile_name = current_time.isoformat().replace(':','_') + '.jpg'
ââââââââfile.filename = file_name
ââââââââ# s3ì ì
ë¡ë
ââââââââ# rekognition ìë¹ì€ë¥Œ ìŽì©íë €ë©Ž,
ââââââââ# 뚌ì s3ì ìŽë¯žì§ íìŒì ì
ë¡ë íŽëìŒ íë€
ââââââââclient = boto3.client('s3',
âââââââââââââââââââââaws_access_key_id = Config.AWS_ACCESS_KEY,
âââââââââââââââââââââaws_secret_access_key = Config.AWS_SECRET_ACCESS_KEY)
ââââââââ
ââââââââtry :
ââââââââââââclient.upload_fileobj(file,
ââââââââââââââââââââââââââââââââââConfig.S3_BUCKET,
ââââââââââââââââââââââââââââââââââfile_name,
ââââââââââââââââââââââââââââââââââExtraArgs = {'ACL' : 'public-read',
âââââââââââââââââââââââââââââââââââââââââââââââ'ContentType':'image/jpeg'})
ââââââââexcept Exception as e:
ââââââââââââreturn {"result":"fail", "error":str(e)}, 500
ââââââââ# 늬ìœê·žëì
ì ìŽì©
ââââââââlabel_list = self.detect_labels(file_name, Config.S3_BUCKET)
ââââââââ
ââââââââreturn {"result":"success", "lable": label_list}
ââââ
ââââdef detect_labels(self, photo, bucket):
ââââââââclient = boto3.client('rekognition',
âââââââââââââââââââââ'ap-northeast-2',
âââââââââââââââââââââaws_access_key_id = Config.AWS_ACCESS_KEY,
âââââââââââââââââââââaws_secret_access_key = Config.AWS_SECRET_ACCESS_KEY)
ââââââââresponse = client.detect_labels(Image={'S3Object':{'Bucket':bucket,'Name':photo}},
ââââââââMaxLabels=10,
ââââââââ# Uncomment to use image properties and filtration settings
ââââââââ#Features=["GENERAL_LABELS", "IMAGE_PROPERTIES"],
ââââââââ#Settings={"GeneralLabels": {"LabelInclusionFilters":["Cat"]},
ââââââââ# "ImageProperties": {"MaxDominantColors":10}}
ââââââââ)
ââââââââprint('Detected labels for ' + photo)
ââââââââprint()
ââââââââprint(response['Labels'])
ââââââââlabel_list = []
ââââââââfor label in response['Labels']:
ââââââââââââprint("Label: " + label['Name'])
ââââââââââââlabel_list.append(label['Name'])
ââââââââreturn label_list
ì¬ì§ ì ë¡ë API í¬ì€ížë§š ìì±
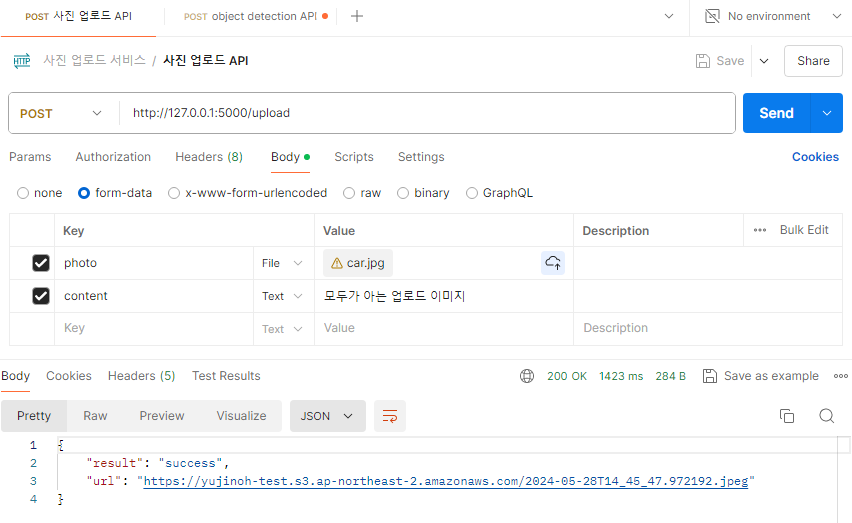
sendíê³ , s3 ë²í·ì ë€ìŽê°ë³Žë©Ž ìëì²ëŒ ì¬ì§ì sendí 목ë¡ë€ìŽ ë¬ë€.
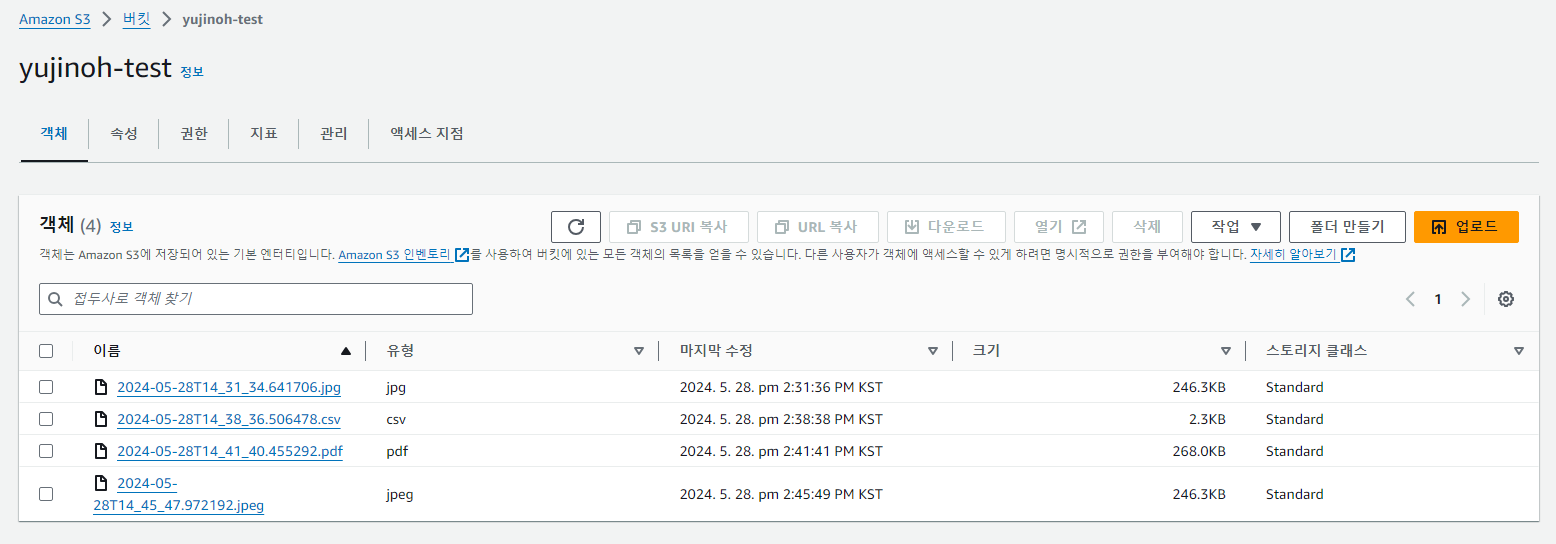
object detection API í¬ì€ížë§š ìì±íê³ sendí멎

vscodeììë ìŽë ê² ë¬ë€.
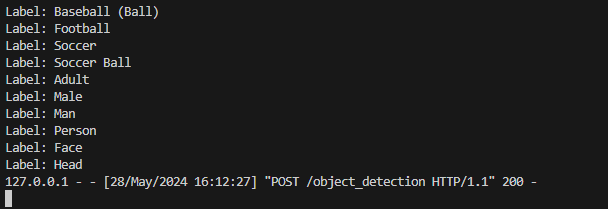
.jpeg, .jpg ë±ì íì ì€ëª ííìŽì§ ë§í¬ìŽë€.
https://developer.mozilla.org/en-US/docs/Web/HTTP/Basics_of_HTTP/MIME_types/Common_types
Common MIME types - HTTP | MDN
This topic lists the most common MIME types with corresponding document types, ordered by their common extensions.
developer.mozilla.org