- install : pip install prophet
- ์ ์๋ฌ ๋ฐ์์ : conda install -c conda-forge prophet
์ํฌํธํ๊ธฐ
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import random
import seaborn as sns
from prophet import Prophet
์๋ณด์นด๋ ๋ฐ์ดํฐ
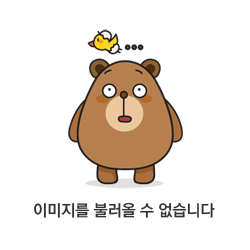
ํ๋กํซ ๋ถ์์ ์ํด, ๋๊ฐ์ ์ปฌ๋ผ๋ง ๊ฐ์ ธ์ค๊ธฐ ('Date', 'AveragePrice')
avocado_prophet_df = df[['Date','AveragePrice']]
avocado_prophet_df
Date | AveragePrice | |
0 | 2015-01-04 | 1.33 |
1 | 2015-01-04 | 1.35 |
2 | 2015-01-04 | 0.93 |
3 | 2015-01-04 | 1.08 |
4 | 2015-01-04 | 1.28 |
... | ... | ... |
7 | 2018-03-25 | 1.63 |
8 | 2018-03-25 | 1.71 |
9 | 2018-03-25 | 1.87 |
10 | 2018-03-25 | 1.93 |
11 | 2018-03-25 | 1.62 |
ds ์ y ๋ก ์ปฌ๋ผ๋ช ์ ํ
avocado_prophet_df.columns = ['ds','y']
avocado_prophet_df
ds | y | |
0 | 2015-01-04 | 1.33 |
1 | 2015-01-04 | 1.35 |
2 | 2015-01-04 | 0.93 |
3 | 2015-01-04 | 1.08 |
4 | 2015-01-04 | 1.28 |
... | ... | ... |
7 | 2018-03-25 | 1.63 |
8 | 2018-03-25 | 1.71 |
9 | 2018-03-25 | 1.87 |
10 | 2018-03-25 | 1.93 |
11 | 2018-03-25 | 1.62 |
ํ๋กํซ ์์ธก
# 1. ๋ผ์ด๋ธ๋ฌ๋ฆฌ๋ฅผ ๋ณ์๋ก ๋ง๋ค๊ณ
prophet = Prophet()
# 2. ๋ฐ์ดํฐ๋ก ํ์ต์ํจ๋ค
prophet.fit(avocado_prophet_df)
# 3. ์์ธกํ๊ณ ์ ํ๋ ๊ธฐ๊ฐ์ ์ ํด์, ๋น์ด์๋ ๋ฐ์ดํฐํ๋ ์์ ๋ง๋ ๋ค
future = prophet.make_future_dataframe(periods=365, freq='D') #365์ผ
# 4. ์์ธก์ ํ๋ค
forecast = prophet.predict(future)
# ์ฐจํธ๋ก ํ์ธ
prophet.plot(forecast)
plt.savefig('chart1.jpg')
plt.show()
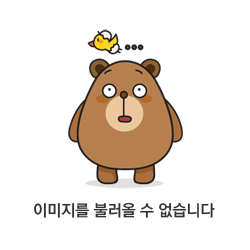
prophet.plot_components(forecast)
plt.savefig('chart2.jpg')
plt.show()
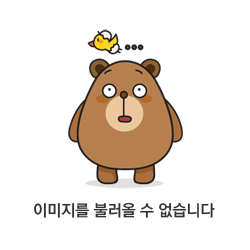
region ์ด west ์ธ ์๋ณด์นด๋์ ๊ฐ๊ฒฉ์ ์์ธกํ์์ค.
df = df[df['region'].str.contains('West')]
df = df.loc[ : , 'Date':'AveragePrice']
import pandas as pd
df = pd.DataFrame(df)
df = df.rename(columns={'Date':'ds', 'AveragePrice':'y'})
prophet = Prophet()
prophet.fit(df)
future = prophet.make_future_dataframe(periods=365, freq='D')
forecast = prophet.predict(future)
prophet.plot(forecast)
plt.show()
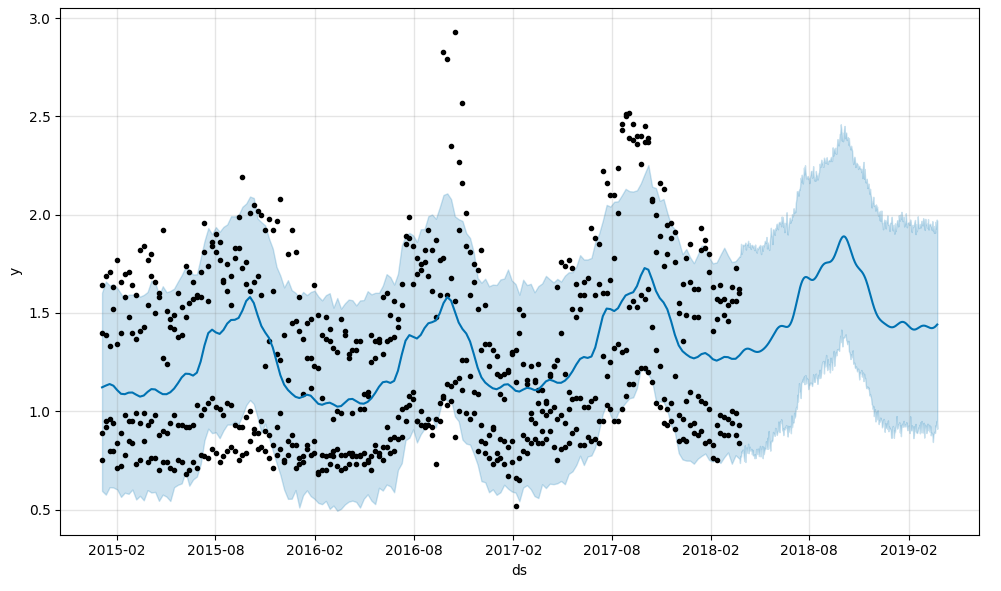
prophet.plot_components(forecast)
plt.show()
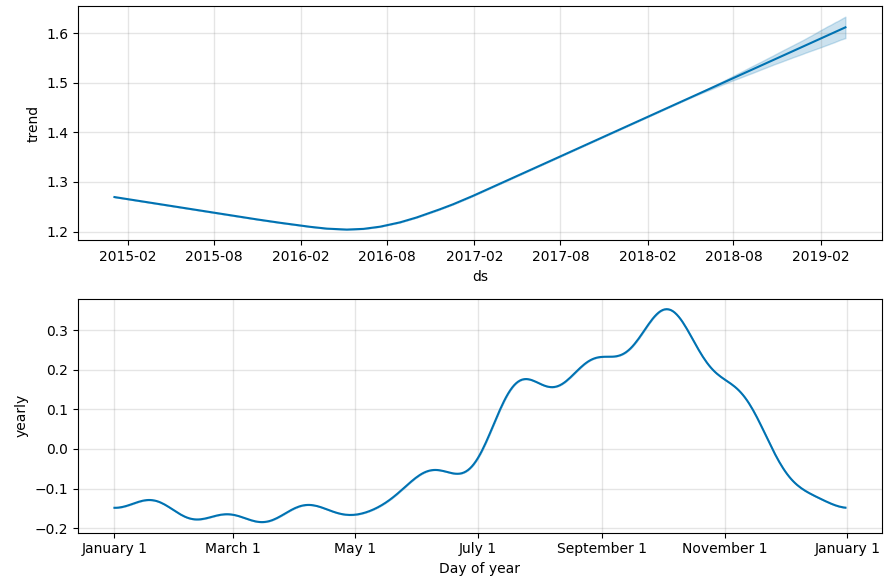
์์นด๊ณ ๋ฒ์ฃ ๋ฐ์ดํฐ
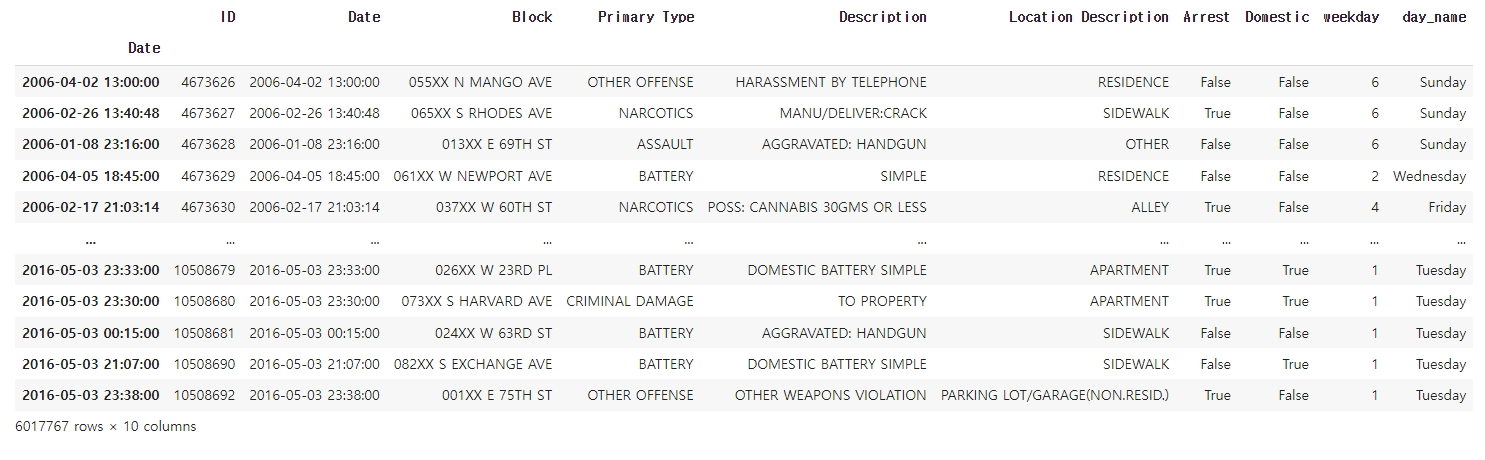
df_prophet = chicago_df.resample('D').size().to_frame().reset_index().rename(columns={'Date':'ds', 0:'y'})
prophet = Prophet()
prophet.fit(df_prophet)
future = prophet.make_future_dataframe(periods=365, freq='D')
forecast = prophet.predict(future)
prophet.plot(forecast)
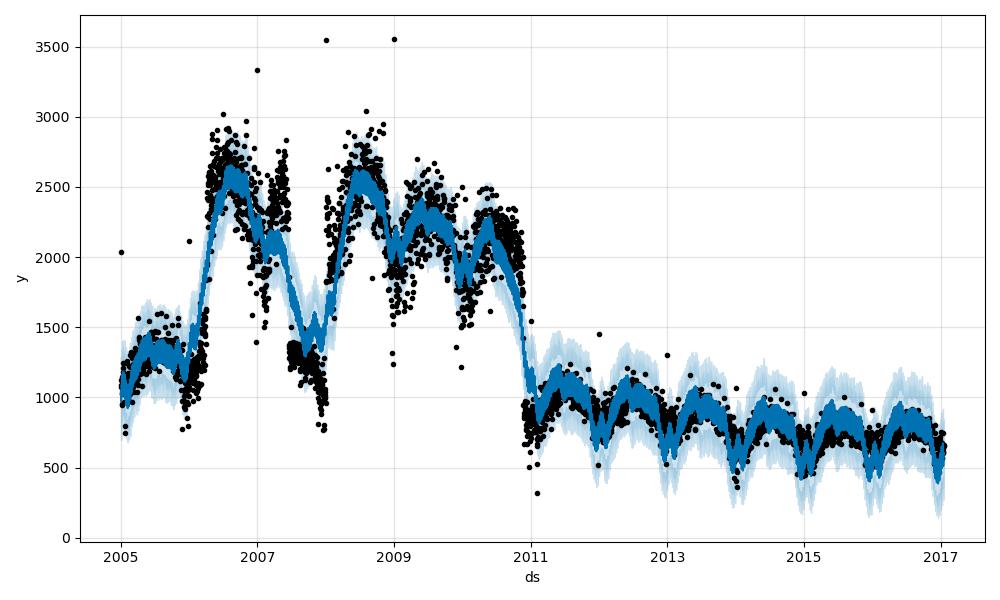
prophet.plot_components(forecast)
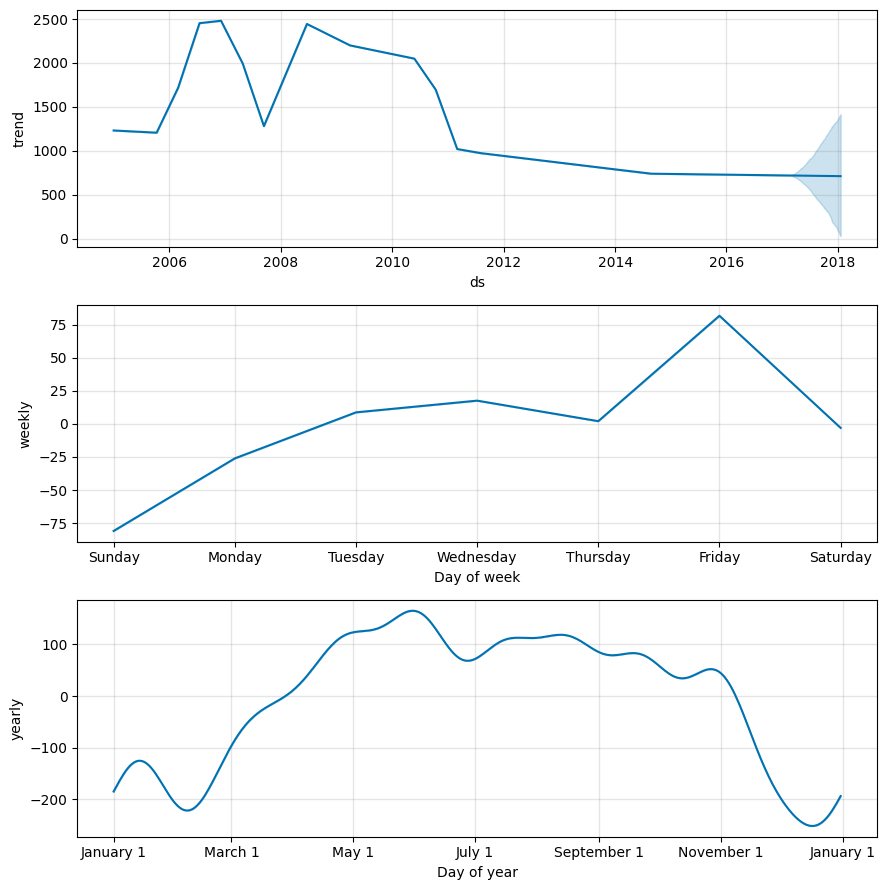